1. 主題: 檔案
1. 打開CodeBlocks
2. 開新Project (Console Application)
3. 把完來的程式碼刪掉
4. 加入新的程式碼
5. 改成以下的程式碼
#include <stdio.h>
FILE * fout=NULL; ///(1)宣告一個檔案指標
int main()
{
fout = fopen("filename.txt","w+"); ///(2)我們要開檔案 ///w+ = read and update
for (int i=0;i<20;i++){
fprintf(fout,"Hello World\n"); ///(3)印出來
}
}
6. 他會自己加一個叫"filename"的檔案 然後在裡面打"Hello World"
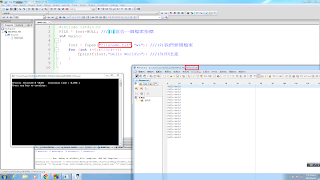
7. 把20個關節的角度印出來 (開一個叫motion的新檔案)
#include <stdio.h>
FILE * fout2=NULL; ///寫另一個檔案
FILE * fin2=NULL; ///讀另一個檔案
float angle[20]; ///有20個關節的角度
int main()
{
fout2=fopen("motion.txt" , "w+");///另一個關節的檔案
for(int i=0;i<20;i++){
fprintf(fout2, "%.2f ", angle[i]); ///把角度印出來
}
}
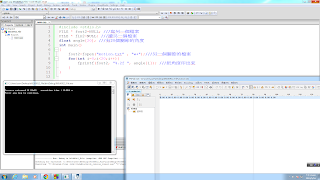
2. 主題: 關節、擺動作
1. 先載入freeglut (跟第一週一樣)
1. Google search "freeglut windows"
2. 從Transmission Zero 下載 "freeglut 3.0.0 MinGW Package"
2. 從Transmission Zero 下載 "freeglut 3.0.0 MinGW Package"
3. 解壓縮後放到桌面
4. 在lib複製一個"libfreeglut.a"命名為"libglut32.a"
5. CodeBlocks 開
6. File -> New -> Project -> GLUT Project
7. 把location改成"C:\Users\user\Desktop\freeglut"
2. 加入程式碼
3. 先做一個茶壺
#include <GL/glut.h>
void display()
{
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
glutSolidTeapot( 0.3 );
glutSwapBuffers();
}
int main(int argc, char**argv)
{
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_DOUBLE | GLUT_DEPTH);
///glutInitWindowSize(600,600);///可以開大一點的window
glutCreateWindow("Week 13 angle motion file");
glutDisplayFunc(display);
glutMainLoop();
}
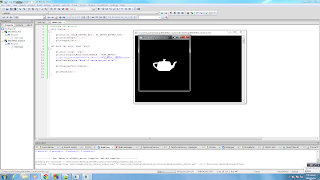
4. 加紅色茶壺
#include <GL/glut.h>
float angle[20];///NOW2
void display()
{
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
glPushMatrix();
glColor3f(1, 1, 1);///white
glutSolidTeapot( 0.3 );///body
glPushMatrix();
glTranslatef(0.3, 0, 0);
glColor3f(1, 0, 0);///red
glutSolidTeapot( 0.2 );///right arm
glPopMatrix();
glPopMatrix();
glutSwapBuffers();
}
int main(int argc, char**argv)
{
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_DOUBLE | GLUT_DEPTH);
///glutInitWindowSize(600,600);///可以開大一點的window
glutCreateWindow("Week 13 angle motion file");
glutDisplayFunc(display);
glutMainLoop();
}
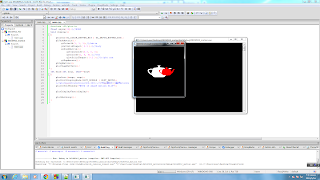
5. 加入旋轉的程式碼(用滑鼠可以轉動)
#include <GL/glut.h>
float angle[20];///NOW2
void display()
{
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
glPushMatrix();
glColor3f(1, 1, 1);///white
glutSolidTeapot( 0.3 );///body
glPushMatrix();
glTranslatef(0.2, 0, 0);///NOW2 (3)掛上去
glRotatef(angle[1], 0,0,1);///NOW2 ///(2)旋轉
glTranslatef(0.15, 0, 0);///NOW2 (1)旋轉中心
glColor3f(1, 0, 0);///red
glutSolidTeapot( 0.2 );///right arm
glPopMatrix();
glPopMatrix();
glutSwapBuffers();
}
int oldX=0;///NOW2
void mouse(int button, int state, int x, int y)///NOW2
{///NOW2
oldX = x;///NOW2
}
void motion(int x, int y)///NOW2
{///NOW2
angle[1] += x-oldX;///NOW2
oldX = x;///NOW2
display();///NOW2
}
int main(int argc, char**argv)
{
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_DOUBLE | GLUT_DEPTH);
///glutInitWindowSize(600,600);///可以開大一點的window
glutCreateWindow("Week 13 angle motion file");
glutMouseFunc(mouse);///NOW2
glutMotionFunc(motion);///NOW2
glutDisplayFunc(display);
glutMainLoop();
}
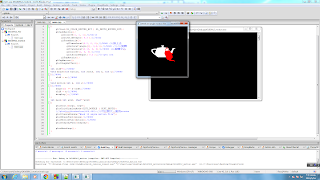
6. 有兩個紅色茶壺(用滑鼠可以轉動)
#include <GL/glut.h>
float angle[20];///NOW2
void display()
{
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
glPushMatrix();
glColor3f(1, 1, 1);///white
glutSolidTeapot( 0.3 );///body
glPushMatrix();
glTranslatef(0.2, 0, 0);///NOW2 (3)掛上去
glRotatef(angle[1], 0,0,1);///NOW2 ///(2)旋轉
glTranslatef(0.15, 0, 0);///NOW2 (1)旋轉中心
glColor3f(1, 0, 0);///red
glutSolidTeapot( 0.2 );///right arm
glPushMatrix();
glTranslatef(0.2, 0, 0);///NOW2 (3)掛上去
glRotatef(angle[1], 0,0,1);///NOW2 ///(2)旋轉
glTranslatef(0.15, 0, 0);///NOW2 (1)旋轉中心
glColor3f(1, 0, 0);///red
glutSolidTeapot( 0.2 );///right lower arm
glPopMatrix();
glPopMatrix();
glPopMatrix();
glutSwapBuffers();
}
int oldX=0;///NOW2
void mouse(int button, int state, int x, int y)///NOW2
{///NOW2
oldX = x;///NOW2
}
void motion(int x, int y)///NOW2
{///NOW2
angle[1] += x-oldX;///NOW2
oldX = x;///NOW2
display();///NOW2
}
int main(int argc, char**argv)
{
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_DOUBLE | GLUT_DEPTH);
///glutInitWindowSize(600,600);///可以開大一點的window
glutCreateWindow("Week 13 angle motion file");
glutMouseFunc(mouse);///NOW2
glutMotionFunc(motion);///NOW2
glutDisplayFunc(display);
glutMainLoop();
}
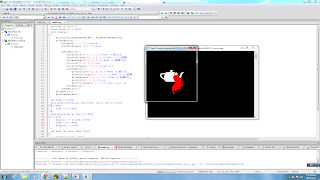
7. 有四個紅色茶壺(用滑鼠可以轉動)
#include <GL/glut.h>
float angle[20];///NOW2
void display()
{
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
glPushMatrix();
glColor3f(1, 1, 1);///white
glutSolidTeapot( 0.3 );///body
glPushMatrix();///右邊
glTranslatef(0.2, 0, 0);///NOW2 (3)掛上去
glRotatef(angle[1], 0,0,1);///NOW2 ///(2)旋轉
glTranslatef(0.15, 0, 0);///NOW2 (1)旋轉中心
glColor3f(1, 0, 0);///red
glutSolidTeapot( 0.2 );///right arm
glPushMatrix();
glTranslatef(0.2, 0, 0);///NOW2 (3)掛上去
glRotatef(angle[1], 0,0,1);///NOW2 ///(2)旋轉
glTranslatef(0.15, 0, 0);///NOW2 (1)旋轉中心
glColor3f(1, 0, 0);///red
glutSolidTeapot( 0.2 );///right lower arm
glPopMatrix();
glPopMatrix();
glPushMatrix();///左邊
glTranslatef(-0.2, 0, 0);///NOW2 (3)掛上去
glRotatef(angle[1], 0,0,1);///NOW2 ///(2)旋轉
glTranslatef(-0.15, 0, 0);///NOW2 (1)旋轉中心
glColor3f(1, 0, 0);///red
glutSolidTeapot( 0.2 );///right arm
glPushMatrix();
glTranslatef(-0.2, 0, 0);///NOW2 (3)掛上去
glRotatef(angle[1], 0,0,1);///NOW2 ///(2)旋轉
glTranslatef(-0.15, 0, 0);///NOW2 (1)旋轉中心
glColor3f(1, 0, 0);///red
glutSolidTeapot( 0.2 );///right lower arm
glPopMatrix();
glPopMatrix();
glPopMatrix();
glutSwapBuffers();
}
int oldX=0;///NOW2
void mouse(int button, int state, int x, int y)///NOW2
{///NOW2
oldX = x;///NOW2
}
void motion(int x, int y)///NOW2
{///NOW2
angle[1] += x-oldX;///NOW2
oldX = x;///NOW2
display();///NOW2
}
int main(int argc, char**argv)
{
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_DOUBLE | GLUT_DEPTH);
///glutInitWindowSize(600,600);///可以開大一點的window
glutCreateWindow("Week 13 angle motion file");
glutMouseFunc(mouse);///NOW2
glutMotionFunc(motion);///NOW2
glutDisplayFunc(display);
glutMainLoop();
}
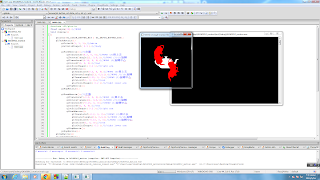
8. 用鍵盤操控要動哪一個關節
#include <GL/glut.h>
float angle[20];///NOW2
int angleID=1;///NOW3
void display()
{
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
glPushMatrix();
glColor3f(1, 1, 1);///white
glutSolidTeapot( 0.3 );///body
glPushMatrix();///右邊
glTranslatef(0.2, 0, 0);///NOW2 (3)掛上去
glRotatef(angle[1], 0,0,1);///NOW2 ///(2)旋轉
glTranslatef(0.15, 0, 0);///NOW2 (1)旋轉中心
glColor3f(1, 0, 0);///red
glutSolidTeapot( 0.2 );///right arm
glPushMatrix();
glTranslatef(0.2, 0, 0);///NOW2 (3)掛上去
glRotatef(angle[2], 0,0,1);///NOW2 ///(2)旋轉
glTranslatef(0.15, 0, 0);///NOW2 (1)旋轉中心
glColor3f(1, 0, 0);///red
glutSolidTeapot( 0.2 );///right lower arm
glPopMatrix();
glPopMatrix();
glPushMatrix();///左邊
glTranslatef(-0.2, 0, 0);///NOW2 (3)掛上去
glRotatef(angle[3], 0,0,1);///NOW2 ///(2)旋轉
glTranslatef(-0.15, 0, 0);///NOW2 (1)旋轉中心
glColor3f(1, 0, 0);///red
glutSolidTeapot( 0.2 );///right arm
glPushMatrix();
glTranslatef(-0.2, 0, 0);///NOW2 (3)掛上去
glRotatef(angle[4], 0,0,1);///NOW2 ///(2)旋轉
glTranslatef(-0.15, 0, 0);///NOW2 (1)旋轉中心
glColor3f(1, 0, 0);///red
glutSolidTeapot( 0.2 );///right lower arm
glPopMatrix();
glPopMatrix();
glPopMatrix();
glutSwapBuffers();
}
int oldX=0;///NOW2
void mouse(int button, int state, int x, int y)///NOW2
{///NOW2
oldX = x;///NOW2
}
#include <stdio.h>///NOW3
void motion(int x, int y)///NOW2
{///NOW2
angle[angleID] += x-oldX;///NOW3
oldX = x;///NOW2
for(int i=0;i<20;i++){///NOW3
printf(" %.2f ", angle[i]);///NOW3
}
printf("\n");///NOW3
display();///NOW2
}
void keyboard(unsigned char key, int x, int y)///NOW3
{///NOW3
if(key=='1') angleID=1;///NOW3
if(key=='2') angleID=2;///NOW3
if(key=='3') angleID=3;///NOW3
if(key=='4') angleID=4;///NOW3
}
int main(int argc, char**argv)
{
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_DOUBLE | GLUT_DEPTH);
///glutInitWindowSize(600,600);///可以開大一點的window
glutCreateWindow("Week 13 angle motion file");
glutKeyboardFunc(keyboard);///NOW3
glutMouseFunc(mouse);///NOW2
glutMotionFunc(motion);///NOW2
glutDisplayFunc(display);
glutMainLoop();
}
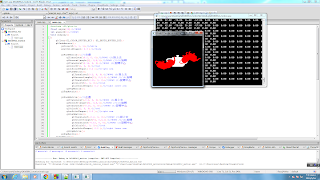
3. 主題: 複習
1. 做一個新的檔案 把角度存起來 (在freeglut的lib裡)
#include <GL/glut.h>
#include <stdio.h>///NOW_FILE (0)
float angle[20];///NOW2
int angleID=1;///NOW3
FILE * fout = NULL;///NOW_FILE (1)
void display()
{
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
glPushMatrix();
glColor3f(1, 1, 1);///white
glutSolidTeapot( 0.3 );///body
glPushMatrix();///右邊
glTranslatef(0.2, 0, 0);///NOW2 (3)掛上去
glRotatef(angle[1], 0,0,1);///NOW2 ///(2)旋轉
glTranslatef(0.15, 0, 0);///NOW2 (1)旋轉中心
glColor3f(1, 0, 0);///red
glutSolidTeapot( 0.2 );///right arm
glPushMatrix();
glTranslatef(0.2, 0, 0);///NOW2 (3)掛上去
glRotatef(angle[2], 0,0,1);///NOW2 ///(2)旋轉
glTranslatef(0.15, 0, 0);///NOW2 (1)旋轉中心
glColor3f(1, 0, 0);///red
glutSolidTeapot( 0.2 );///right lower arm
glPopMatrix();
glPopMatrix();
glPushMatrix();///左邊
glTranslatef(-0.2, 0, 0);///NOW2 (3)掛上去
glRotatef(angle[3], 0,0,1);///NOW2 ///(2)旋轉
glTranslatef(-0.15, 0, 0);///NOW2 (1)旋轉中心
glColor3f(1, 0, 0);///red
glutSolidTeapot( 0.2 );///right arm
glPushMatrix();
glTranslatef(-0.2, 0, 0);///NOW2 (3)掛上去
glRotatef(angle[4], 0,0,1);///NOW2 ///(2)旋轉
glTranslatef(-0.15, 0, 0);///NOW2 (1)旋轉中心
glColor3f(1, 0, 0);///red
glutSolidTeapot( 0.2 );///right lower arm
glPopMatrix();
glPopMatrix();
glPopMatrix();
glutSwapBuffers();
}
int oldX=0;///NOW2
void mouse(int button, int state, int x, int y)///NOW2
{///NOW2
oldX = x;///NOW2
}
void motion(int x, int y)///NOW2
{///NOW2
angle[angleID] += x-oldX;///NOW3
oldX = x;///NOW2
if(fout == NULL) fout = fopen("motion.txt", "w+");///NOW_FILE (2)
for(int i=0;i<20;i++){///NOW3
printf(" %.2f ", angle[i]);///NOW3
fprintf(fout, " %.2f ", angle[i]);///NOW_FILE
}
printf("\n");///NOW3
fprintf(fout, "\n");///NOW_FILE
display();///NOW2
}
void keyboard(unsigned char key, int x, int y)///NOW3
{///NOW3
if(key=='1') angleID=1;///NOW3
if(key=='2') angleID=2;///NOW3
if(key=='3') angleID=3;///NOW3
if(key=='4') angleID=4;///NOW3
if(key=='w'){///NOW_FILE
fout = fopen("motion.txt", "w+");///NOW_FILE (2)
}
}
int main(int argc, char**argv)
{
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_DOUBLE | GLUT_DEPTH);
///glutInitWindowSize(600,600);///可以開大一點的window
glutCreateWindow("Week 13 angle motion file");
glutKeyboardFunc(keyboard);///NOW3
glutMouseFunc(mouse);///NOW2
glutMotionFunc(motion);///NOW2
glutDisplayFunc(display);
glutMainLoop();
}
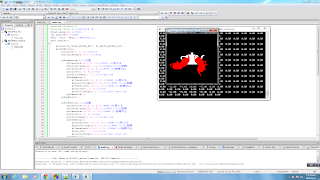
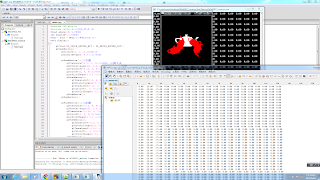
2. 做一個新的檔案 把角度存起來 (在freeglut的lib裡)
#include <GL/glut.h>
#include <stdio.h>///NOW_FILE (0)
float angle[20];///NOW2
int angleID=1;///NOW3
FILE * fout = NULL;///NOW_FILE (1)
FILE * fin = NULL;///NOW_FILE_READ (1)
void display()
{
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
glPushMatrix();
glColor3f(1, 1, 1);///white
glutSolidTeapot( 0.3 );///body
glPushMatrix();///右邊
glTranslatef(0.2, 0, 0);///NOW2 (3)掛上去
glRotatef(angle[1], 0,0,1);///NOW2 ///(2)旋轉
glTranslatef(0.15, 0, 0);///NOW2 (1)旋轉中心
glColor3f(1, 0, 0);///red
glutSolidTeapot( 0.2 );///right arm
glPushMatrix();
glTranslatef(0.2, 0, 0);///NOW2 (3)掛上去
glRotatef(angle[2], 0,0,1);///NOW2 ///(2)旋轉
glTranslatef(0.15, 0, 0);///NOW2 (1)旋轉中心
glColor3f(1, 0, 0);///red
glutSolidTeapot( 0.2 );///right lower arm
glPopMatrix();
glPopMatrix();
glPushMatrix();///左邊
glTranslatef(-0.2, 0, 0);///NOW2 (3)掛上去
glRotatef(angle[3], 0,0,1);///NOW2 ///(2)旋轉
glTranslatef(-0.15, 0, 0);///NOW2 (1)旋轉中心
glColor3f(1, 0, 0);///red
glutSolidTeapot( 0.2 );///right arm
glPushMatrix();
glTranslatef(-0.2, 0, 0);///NOW2 (3)掛上去
glRotatef(angle[4], 0,0,1);///NOW2 ///(2)旋轉
glTranslatef(-0.15, 0, 0);///NOW2 (1)旋轉中心
glColor3f(1, 0, 0);///red
glutSolidTeapot( 0.2 );///right lower arm
glPopMatrix();
glPopMatrix();
glPopMatrix();
glutSwapBuffers();
}
int oldX=0;///NOW2
void mouse(int button, int state, int x, int y)///NOW2
{///NOW2
oldX = x;///NOW2
}
void motion(int x, int y)///NOW2
{///NOW2
angle[angleID] += x-oldX;///NOW3
oldX = x;///NOW2
if(fout == NULL) fout = fopen("motion.txt", "w+");///NOW_FILE (2)
for(int i=0;i<20;i++){///NOW3
printf(" %.2f ", angle[i]);///NOW3
fprintf(fout, " %.2f ", angle[i]);///NOW_FILE
}
printf("\n");///NOW3
fprintf(fout, "\n");///NOW_FILE
display();///NOW2
}
void keyboard(unsigned char key, int x, int y)///NOW3
{///NOW3
if(key=='1') angleID=1;///NOW3
if(key=='2') angleID=2;///NOW3
if(key=='3') angleID=3;///NOW3
if(key=='4') angleID=4;///NOW3
if(key=='w'){///NOW_FILE
fout = fopen("motion.txt", "w+");///NOW_FILE (2)
}
if(key=='r'){
if(fin==NULL) fin = fopen("motion.txt", "r");///NOW_FILE_READ (2)
///scanf("%d", &n);
for(int i=0;i<20; i++){///NOW_FILE_READ
fscanf(fin, "%f", &angle[i]);///NOW_FILE_READ
printf(" %.2f ", angle[i]);///順便印出來看數字對不對
}
}
glutPostRedisplay();///NOW_FILE_READ 和 display()很像
}
int main(int argc, char**argv)
{
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_DOUBLE | GLUT_DEPTH);
///glutInitWindowSize(600,600);///可以開大一點的window
glutCreateWindow("Week 13 angle motion file");
glutKeyboardFunc(keyboard);///NOW3
glutMouseFunc(mouse);///NOW2
glutMotionFunc(motion);///NOW2
glutDisplayFunc(display);
glutMainLoop();
}
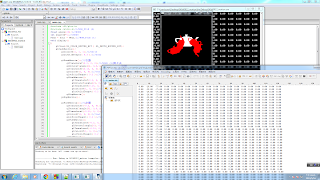
3. 按R就可以讀取檔案(會自己一筆一筆跑)(自己動)
#include <GL/glut.h>
#include <stdio.h>///NOW_FILE (0)
float angle[20];///NOW2
int angleID=1;///NOW3
FILE * fout = NULL;///NOW_FILE (1)
FILE * fin = NULL;///NOW_FILE_READ (1)
void display()
{
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
glPushMatrix();
glColor3f(1, 1, 1);///white
glutSolidTeapot( 0.3 );///body
glPushMatrix();///右邊
glTranslatef(0.2, 0, 0);///NOW2 (3)掛上去
glRotatef(angle[1], 0,0,1);///NOW2 ///(2)旋轉
glTranslatef(0.15, 0, 0);///NOW2 (1)旋轉中心
glColor3f(1, 0, 0);///red
glutSolidTeapot( 0.2 );///right arm
glPushMatrix();
glTranslatef(0.2, 0, 0);///NOW2 (3)掛上去
glRotatef(angle[2], 0,0,1);///NOW2 ///(2)旋轉
glTranslatef(0.15, 0, 0);///NOW2 (1)旋轉中心
glColor3f(1, 0, 0);///red
glutSolidTeapot( 0.2 );///right lower arm
glPopMatrix();
glPopMatrix();
glPushMatrix();///左邊
glTranslatef(-0.2, 0, 0);///NOW2 (3)掛上去
glRotatef(angle[3], 0,0,1);///NOW2 ///(2)旋轉
glTranslatef(-0.15, 0, 0);///NOW2 (1)旋轉中心
glColor3f(1, 0, 0);///red
glutSolidTeapot( 0.2 );///right arm
glPushMatrix();
glTranslatef(-0.2, 0, 0);///NOW2 (3)掛上去
glRotatef(angle[4], 0,0,1);///NOW2 ///(2)旋轉
glTranslatef(-0.15, 0, 0);///NOW2 (1)旋轉中心
glColor3f(1, 0, 0);///red
glutSolidTeapot( 0.2 );///right lower arm
glPopMatrix();
glPopMatrix();
glPopMatrix();
glutSwapBuffers();
}
int oldX=0;///NOW2
void mouse(int button, int state, int x, int y)///NOW2
{///NOW2
oldX = x;///NOW2
}
void motion(int x, int y)///NOW2
{///NOW2
angle[angleID] += x-oldX;///NOW3
oldX = x;///NOW2
if(fout == NULL) fout = fopen("motion.txt", "w+");///NOW_FILE (2)
for(int i=0;i<20;i++){///NOW3
printf(" %.2f ", angle[i]);///NOW3
fprintf(fout, " %.2f ", angle[i]);///NOW_FILE
}
printf("\n");///NOW3
fprintf(fout, "\n");///NOW_FILE
display();///NOW2
}
void keyboard(unsigned char key, int x, int y)///NOW3
{///NOW3
if(key=='1') angleID=1;///NOW3
if(key=='2') angleID=2;///NOW3
if(key=='3') angleID=3;///NOW3
if(key=='4') angleID=4;///NOW3
if(key=='w'){///NOW_FILE
fout = fopen("motion.txt", "w+");///NOW_FILE (2)
}
if(key=='r'){
if(fin==NULL) fin = fopen("motion.txt", "r");///NOW_FILE_READ (2)
///scanf("%d", &n);
for(int i=0;i<20; i++){///NOW_FILE_READ
fscanf(fin, "%f", &angle[i]);///NOW_FILE_READ
printf(" %.2f ", angle[i]);///順便印出來看數字對不對
}
}
glutPostRedisplay();///NOW_FILE_READ 和 display()很像
}
int main(int argc, char**argv)
{
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_DOUBLE | GLUT_DEPTH);
///glutInitWindowSize(600,600);///可以開大一點的window
glutCreateWindow("Week 13 angle motion file");
glutKeyboardFunc(keyboard);///NOW3
glutMouseFunc(mouse);///NOW2
glutMotionFunc(motion);///NOW2
glutDisplayFunc(display);
glutMainLoop();
}
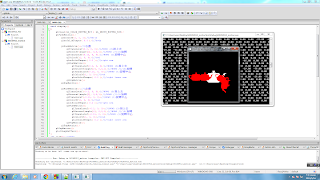
4. 作業: 機器人擺動作
沒有留言:
張貼留言